【C++】常見題目解答
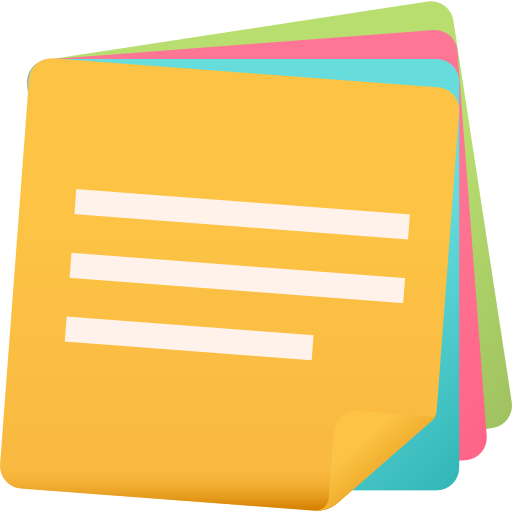
文章目錄
最後更新:10/6 我整理出C++題目常見的題目並寫出我認為的最佳解(取自西苑高一電腦課)。也有提供一些減少程式碼的常用技巧。如果解不出來到想砸電腦或想只到更簡單的方法可以來看看
網站終於搶救成功啦!這兩天來更新!這裡的程式僅供參考,請不要偷懶直接複製貼上,小考你不會過的。
關於有人問我是誰,選單有各種連結。
目前更新到503 上課只會上到425
小提示
當然如果你只是想看解答然後像月同學一樣手機開超大聲打音遊可以點我跳到解答
如果你忘記C語言的語法想看看可以閱讀這篇文章,不過相信你學過C++就不會想要碰它了。
減少程式碼的常用技巧
這裡講的是真的少打一點,不是全部縮成一排。減少程式碼可以方便閱讀且在bebug(找錯誤)比較方便
定義時賦予值
1int a b;
2a=87;
可以簡化成int a=87, b;
一行
在輸出行內進行運算
1a=x+y;
2cout<<a
可以簡化成cout<<x+y;
一行
if else省去大括號
if else裡面如果只有一行指令可以省去大括號{}
1if(a>b){
2 cout<<a;
3}else{
4 cout<<b
5}
可以簡化成if(a>b) cout<<a; else cout<<b;
一行
數學含式庫<math.h>
列出來怕你忘記
M_E
回傳自然常數 eM_PI
回傳圓周率 πM_SQRT2
回傳根號2sin(x)
cos(x)
tan(x)
asin(x)
acos(x)
atan(x)
不解釋bj4exp(x)
回傳自然常數 e 的x
次方pow(x, y)
回傳x
的y
次方pow(x)
回傳10的x
次方sqrt(x)
回傳x
的根號log(x)
回傳以e
為底的對數log10(x)
回傳以10為底的對數abs(x)
回傳整數x
的絕對值fabs(x)
回傳實數x
的絕對值
題目解答
就..雖然我很貼心還給你了複製鍵...但有問題要問...
裡面的換行空格是大家的習慣寫法,可以參考。且你會發現雖然空格多一點程式還是比標準答案少
裡面有一些東西還沒有教過,有興趣可以問我或是Google。
目前只更新到308
Ch.1
101.Hello C++
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "Hello C++";
6 return 0;
7}
102.BMI
1#include<iostream>
2
3using namespace std;
4int main() {
5 float weight, height, BMI;
6 cin >> weight >> height;
7 BMI = weight / ((height / 100) * (height / 100));
8 cout << "BMI為" << BMI;
9 return 0;
10}
Ch.2
201.運算子
先乘除後加減(依照優先順序)
- 有括弧的先計算
- 多個括弧皆使用小括弧。EX:((8+9)-7)
- 整數跟整數相除的結果為整數
運算子 | 定義 | 優先順序 | 結合律 |
---|---|---|---|
++/-- | 後置遞增/遞減 | 2 | 左 |
++/-- | 前置遞增/遞減 | 3 | 右 |
+/- | 正負號 | 3 | 右 |
* | 乘法 | 5 | 左 |
/ | 除法 | 5 | 左 |
% | 餘數(mod) | 5 | 左 |
+/- | 加法/減法 | 6 | 左 |
1#include<iostream>
2
3using namespace std;
4int main() {
5 int x, y;
6 cin >> x >> y;
7 cout << x << " 加 " << y << " 的和是 " << x + y << endl;
8 cout << x << " 減 " << y << " 的差是 " << x - y << endl;
9 cout << x << " 乘 " << y << " 的積是 " << x * y << endl;
10 cout << x << " 除 " << y << " 的結果是 " << 1.0 * x / y << endl;
11 cout << x << " 除 " << y << " 的商是 " << x / y << endl;
12 cout << x << " 除 " << y << " 的餘數是 " << x % y << endl;
13 return 0;
14}
202.成績計算
請製作一個程式,輸入五個成績,計算總和與平均並輸出
C++換行可以用<<endl
,但C語言的\n
明顯短了三倍
1#include<iostream>
2
3using namespace std;
4int main() {
5 float sum, a, b;
6 for (int i = 1; i < 6; ++i) {
7 cin >> b;
8 a += b;
9 }
10 cout << "總和:" << a;
11 cout << "\n平均:" << a / 5;
12 return 0;
13}
203.矩形周長面積
請製作一個程式,輸入長方形的長 寬,並計算周長與面積
1#include<iostream>
2
3using namespace std;
4int main() {
5 int x, y;
6 cin >> x >> y;
7 cout << "周長=" << 2 * (x + y);
8 cout << "\n面積=" << x * y;
9 return 0;
10}
204.畢氏定理
請製作一個程式,輸入直角三角形兩邊長,利用畢氏定理求斜邊長
sqrt(x)
回傳 x
的平方根(Square Root)
1#include<iostream>
2#include<cmath>
3
4using namespace std;
5int main() {
6 float x, y;
7 cin >> x >> y;
8 cout << "斜邊長=" << sqrt(x * x + y * y);
9 return 0;
10}
205.兩點距離
請製作一個程式,輸入二維中兩點座標,計算出兩點距離
pow(x, y)
回傳 x
的 y
次方
1#include<iostream>
2
3#include<cmath>
4
5using namespace std;
6int main() {
7 float x1, x2, y1, y2;
8 cin >> x1 >> y1 >> x2 >> y2;
9 cout << "兩點距離=" << sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
10 return 0;
11}
206.兩點求一線
請製作一個程式,輸入二維中兩點座標,計算出通過兩點的直線方程式
1#include<iostream>
2#include<cmath>
3
4using namespace std;
5int main(){
6 float x1,x2,y1,y2,m;
7 cin>>x1>>y1>>x2>>y2;
8 cout<<"方程式:y="<<(y1-y2)/(x1-x2)<<"x+"<<(y1*x2-y2*x1)/(x2-x1);
9}
斜率m
為y變化量除以x變化量。將一數代數y=mx+b
即可求出b並寫出方程式。b
只會用一次算出來直接輸出,不用多設一個變數。
不過浮點數是使用科學記號儲存,有一定的誤差 當斜率本身已經有誤差時,再用來算出截距,誤差將擴大。因此最後一個測資會有誤差。
1#include<iostream>
2#include<cmath>
3using namespace std;
4int main(){
5 float x1,x2,y1,y2,m;
6 cin>>x1>>y1>>x2>>y2;
7 m=(y1-y2)/(x1-x2);
8 cout << "方程式:y=" << m << "x+" << y2-x2*m;
9}
Ch.3
301.奇偶同籠?
請製作一個程式,可以讓使用者輸入一個整數,判斷是奇數還是偶數
如果否則的語法是
1if (條件式){
2 程式區塊;
3} else {
4 程式區塊;
5}
如果大括號裡面只有一行程式可以省略
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a;
6 cin >> a;
7 if (a % 2 == 0) cout << "偶數";
8 else cout << "奇數";
9 return 0;
10}
302.及格?
請製作一個程式,讓使用者輸入一個成績,顯示「成績及格」或「成績不及格」
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a;
6 cin >> a;
7 if (a >= 60) cout << "成績及格";
8 else cout << "成績不及格";
9 return 0;
10}
303.幾科不及格
請製作一個程式:
- 可以輸入五個成績
- 計算有幾科不及格
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a[5], b = 0;
6 cin >> a[0] >> a[1] >> a[2] >> a[3] >> a[4];
7 for (int y: a)
8 if (y < 60) b++;
9 cout << "共" << b << "科不及格";
10 return 0;
11}
304.明年當學弟/妹?
請製作一個程式:
- 可以輸入五個成績
- 判斷明年是否當學弟妹(不及格科數達一半)
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a[5], b = 0;
6 cin >> a[0] >> a[1] >> a[2] >> a[3] >> a[4];
7 for (int y: a)
8 if (y < 60) b++;
9 if (b > 2) cout << "明年當學弟妹";
10 else cout << "明年當學長姊";
11 return 0;
12}
305.比大小
請製作一個程式,輸入兩個正整數,輸出較大者
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b;
6 cin >> a >> b;
7 if (a > b) cout << a;
8 else cout << b;
9 return 0;
10}
306.本丸好呷
某數字商店飯糰第二件59折,以低價者計。請製作一個程式,輸入兩個正整數,代表兩顆飯糰的定價,請計算優惠後的總價。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b, c;
6 cin >> a >> b;
7 if (a > b) c = 0.59 * b + a;
8 else c = 0.59 * a + b;
9 cout << "優惠價=" << c;
10 return 0;
11}
307.比仨大
請製作一個程式,輸入三個正整數,輸出最大者
更新:最大值b如果一開始沒有給定值會被設為0。故先將b設為第一個數再進行比較。
1#include<iostream>
2using namespace std;
3int main(){
4 int a[3];
5 cin>>a[0]>>a[1]>>a[2];
6 int b = a[0];
7 for( int y : a ) if(y>b) b=y;
8 cout<<"最大值="<<b;
9 return 0;
10}
308.比不完
請製作一個程式,輸入五個正整數,輸出最大者
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a[5], b;
6 cin >> a[0] >> a[1] >> a[2] >> a[3] >> a[4];
7 for (int y: a)
8 if (y > b) b = y;
9 cout << "最大值=" << b;
10 return 0;
11}
309.正負?
請製作一個程式,判斷輸入的數字是正數、負數還是0
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a;
6 cin >> a;
7 if (a > 0) cout << "正數";
8 else if (a == 0) cout << "零";
9 else cout << "負數";
10 return 0;
11}
310.分級
請製作一個程式,讓使用者輸入成績,並判斷等級:
- 90~100分:A
- 80~89分:B
- 70~79分:C
- 60~69分:D
- 0~59分:F
1#include<iostream>
2using namespace std;
3int main() {
4 int a;
5 cin >> a;
6 switch (a / 10) {
7 case 10:
8 case 9:
9 cout << "A";
10 break;
11 case 8:
12 cout << "B";
13 break;
14 case 7:
15 cout << "C";
16 break;
17 case 6:
18 cout << "D";
19 break;
20 default:
21 cout << "F";
22 }
23 return 0;
24}
311.電腦教室不能帶飲料進來
某項數字商店飲料定價87元,第二件8折,第三件77折 請製作一個程式可以輸入件數計算價錢
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a,c;
6 float b[4]={0,1,1.8,2.57};
7 cin >> a;
8 c=a/3*223.59+b[a%3]*87;
9 cout << "總價=" << c;
10 return 0;
11}
312.一塊錢玩兩次
ax2 + bx + c = 0,請製作一個程式,輸入a、b、c,解一元二次方程式。
若a = 0則輸出ERROR
令d = b2 - 4ac:
若d = 0,則輸出方程式唯一解x=-b/2a
若d > 0,則輸出方程式兩解x=(-b±√d)/2a
若d < 0,則輸出無實數解
1#include<iostream>
2
3#include<cmath>
4
5using namespace std;
6int main() {
7 float a, b, c;
8 cin >> a >> b >> c;
9 if (a == 0) cout << "ERROR";
10 else {
11 float d = b * b - 4 * a * c;
12 if (d < 0) cout << "無實數解";
13 else {
14 if (d == 0) cout << "唯一解x=" << 0 - b / (a * 2);
15 else cout << "兩解:\nx1=" << (0 - b + sqrt(d)) / 2 * a << "\nx2=" << (0 - b - sqrt(d)) / 2 * a;
16 }
17 }
18 return 0;
19}
313.三角形
請製作一個程式,輸入三角形三邊長a、b、c,先判斷能否構成三角形,若無法構成三角形則輸出ERROR,然後評定三角形種類,最後計算三角形面積。
- 假如𝑎2+𝑏2>𝑐2,為銳角三角形
- 假如𝑎2+𝑏2=𝑐2,為直角三角形
- 假如𝑎2+𝑏2<𝑐2,為鈍角三角形
- 令d=(a+b+c)/2,三角形面積=√(d(d−a)(d−b)(d−c))
1#include<iostream>
2
3#include<cmath>
4
5using namespace std;
6int main() {
7 float a, b, c, d;
8 cin >> a >> b >> c;
9 if (a > b) {
10 d = a;
11 a = b;
12 b = d;
13 }
14 if (b > c) {
15 d = b;
16 b = c;
17 c = d;
18 }
19 if (c >= a + b) {
20 cout << "ERROR";
21 } else {
22
23 int e = b * b + a * a;
24 int f = c * c;
25 if (e > f) cout << "銳";
26 else
27 if (e == f) cout << "直";
28 else cout << "鈍";
29 float D = (a + b + c) / 2;
30 cout << "角三角形\n面積=" << sqrt(D * (D - a) * (D - b) * (D - c));
31 }
32 return 0;
33}
Ch.4
這一段有點尷尬,沒有人叫你用for
迴圈你幹嘛寫那麼多行跑那麼久?測資要什麼複製貼上就好了。
如果你想用正規的辦法for
的原型在這裡相信你會的uwu
1for(變數設為;如果...; 變數要...){
2 指令區塊;
3 [break;] //跳出迴圈
4 [continue;] //跳下一次迴圈
5}
比如說這兩個程式都會輸出uwu uwu uwu
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i;
6 for (i = 0; i < a; i++) {
7 cout << "uwu ";
8 }
9 return 0;
10}
1#include<iostream>
2
3using namespace std;
4int main() {
5 for (int i = 0; i < a; i++)
6 cout << "uwu ";
7 return 0;
8}
401
請列印出1 2 3 4 5 6 7 8 ......19
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19";
6 return 0;
7}
402
請列印出1 3 5 7 9 11 13 15 17 19
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "1 3 5 7 9 11 13 15 17 19 ";
6 return 0;
7}
403
請列印出7 6 5 4 3 2 1 0 -1 -2 -3
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "7 6 5 4 3 2 1 0 -1 -2 -3 ";
6 return 0;
7}
405
請列印出7 5 3 1 -1 -3 -5 -7 -9 -11
不要問我404去哪。404找不到此頁面
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "7 5 3 1 -1 -3 -5 -7 -9 -11 ";
6 return 0;
7}
406
請製作一個程式計算1~100之和
國小數學會吧...梯形公式。當然你要直接輸出5050也可以
1#include<iostream>
2
3using namespace std;
4int main() {
5 cout << "5050";
6 return 0;
7}
407
輸入兩個整數a,b,計算a到b的合(包含a、b)
例如輸入1跟100,計算1~100之合為5050
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b;
6 cin >> a >> b;
7 cout << (a + b) * (abs(a - b) + 1) / 2;
8 return 0;
9
10}
408
請製作一個程式,輸入的第一個正整數為班級人數n,代表接下來會有n個正整數,為班上每個人的成績。請計算班級總分。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b, c = 0, i;
6 cin >> a;
7 for (i = 0; i < a; i++) {
8 cin >> b;
9 c = c + b;
10 }
11 cout << c;
12 return 0;
13}
409
請製作一個程式,輸入的第一個正整數為班級人數n,代表接下來會有n個正整數,為班上每個人的成績。請計算班級平均。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b, c = 0, i;
6 cin >> a;
7 for (i = 0; i < a; i++) {
8 cin >> b;
9 c = c + b;
10 }
11 cout << 1.0 * c / a;
12 return 0;
13}
410
請製作一個程式,輸入的第一個正整數為班級人數n,代表接下來會有n個正整數,為班上每個人的成績。請計算班級中不及格的人數。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int n,a,b=0,i;
6 cin >> n;
7 for (i = 0; i < n; i++) {
8 cin >> a;
9 if(a<60) b++;
10 }
11 cout << b;
12 return 0;
13}
411
請製作一個程式,將1~548701487的整數中篩選出94或87的倍數,
- 計算有多少個
- 計算這些數的總和
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i, a = 0, b = 0;
6 for (i = 1; i <= 548701487; i++) {
7 if (i % 87 == 0 || i % 94 == 0) {
8 a++;
9 b += i;
10 }
11 }
12 cout << a << endl << b;
13 return 0;
14}
412
請製作一個程式,輸入一個整數n,計算1~n的整數中(n<10000),出現過多少次5
例如2345含有一次5,5555含有四次5
1#include<iostream>
2
3#include<math.h>
4
5using namespace std;
6int main() {
7 int i, o, a, b = 0, c;
8 cin >> a;
9 for (i = 1; i <= a; i++) {
10 for (o = 0; o <= 5; o++) {
11 c = i / pow(10, o);
12 if (c % 10 == 5) b++;
13 }
14 }
15 cout << b;
16 return 0;
17}
413
請製作一個程式,輸入一個整數x,再輸入一個整數y,檢查y是否為x的因數,輸出1或0
1#include<iostream>
2using namespace std;
3int main() {
4 int a,b;
5 cin>>a>>b;
6 if(a%b==0) cout<<1; else cout<<0;
7 return 0;
8}
414
請製作一個程式,輸入一個整數n,求其所有因數
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i,a;
6 cin>>a;
7 for (i = 1; i <= a; i++) {
8 if(a%i==0) cout << i<<" ";
9
10 }
11 return 0;
12}
415
請製作一個程式,輸入一個整數,求因數數量
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i,a,b=0;
6 cin>>a;
7 for (i = 1; i <= a; i++)
8 if(a%i==0) b++;
9 cout<<b;
10 return 0;
11}
416
請製作一個程式,輸入一個整數,判斷其是質數還是合數
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i,a,b=0;
6 cin>>a;
7 for (i = 1; i <= a; i++)
8 if(a%i==0) b++;
9if(b==2) cout<<"質數"; else
10 cout<<"合數";
11 return 0;
12}
417
請製作一個程式,輸入一個整數n,列出費式數列n項
1 1 2 3 5 8 13 21……
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, b = 0, x = 0, y = 1, z, o;
6 cin >> a;
7 for (o = 0; o < a; o++) {
8 z = x;
9 x = x + y;
10 y = z;
11 cout << x << " ";
12 }
13
14 return 0;
15}
418
請製作一個程式,使用者輸入兩個數字,使用輾轉相除法,求兩數最大公因數
1#include<iostream>
2using namespace std;
3
4int main()
5{
6 int a, b, t;
7
8 while( cin >> a >> b )
9 {
10 while( b!=0 )
11 {
12 t = b;
13 b = a%b;
14 a = t;
15 }
16 cout << a << endl;
17 }
18
19 return 0;
20}
419
請製作一個程式,使用者輸入一個整數n,求質因數分解
1#include<iostream>
2using namespace std;
3
4int main()
5{
6 int n,i;
7 cin >> n;
8 i = 2;
9 while( n > 1 ){
10 while( n%i == 0 ){
11 cout << i;
12 n = n/i;
13 if(n!=1) cout<<"*";
14 }
15 i = i+1;
16 }
17 cout << endl;
18
19 return 0;
20}
420
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的星星直角三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8 for (int i = 0; i <= n; i++) {
9 for (int o = 0; o < i; o++) cout << "*";
10 cout << endl;
11 }
12
13 return 0;
14}
421
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的反星星直角三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8
9 for (int i = 0; i <= n; i++) {
10 for (int a = 0; a < i; a++) cout << " ";
11 for (int o = 0; o < n - i; o++) cout << "*";
12 cout << endl;
13 }
14
15 return 0;
16}
422
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的數字三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8 for (int i = 0; i <= n; i++) {
9 for (int o = 1; o <= i; o++) cout << o;
10 cout << endl;
11 }
12
13 return 0;
14}
423
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的數字三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8 for (int i = 0; i <= n; i++) {
9 for (int o = n; o > 0; o--)
10 if (o > i) cout << o;
11 cout << endl;
12 }
13
14 return 0;
15}
424
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的數字三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8 for (int i = 0; i <= n; i++) {
9 for (int o = 1; o <= n; o++)
10 if (o > n - i) cout << o;
11 cout << endl;
12 }
13
14 return 0;
15}
425
請製作一個程式,輸入一個正整數n,列印出底為n、高為n的數字三角形
1#include<iostream>
2
3using namespace std;
4
5int main() {
6 int n;
7 cin >> n;
8 for (int i = 0; i <= n; i++) {
9 for (int o = 1; o <= n; o++)
10 if (o > n - i) cout << o;
11 cout << endl;
12 }
13
14 return 0;
15}
426
請製作一個程式,輸入一個整數n,列印出2~n之間的所有質數(包含2、n)
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i, a, b = 0;
6 cin >> a;
7 for (int o = 2; o <= a; o++) {
8 b = 0;
9 for (i = 2; i < o; i++)
10 if (o % i == 0) b = 1;
11 if (b == 0) cout << o << " ";
12 }
13 return 0;
14}
這些應該夠你們用幾週了,剩下的有空再繼續更新。
501
請製作一個程式,輸入一個正整數n,代表接下來共有n個整數,計算其總合
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i, a, b, c = 0;
6 cin >> a;
7 for (i = 0; i < a; i++) {
8 cin >> b;
9 c += b;
10 }
11 cout << c;
12 return 0;
13}
502
請製作一個程式,輸入一個正整數n,代表接下來共有n個整數,請輸出最大值
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i, a, b, c = -100;
6 cin >> a;
7 for (i = 0; i < a; i++) {
8 cin >> b;
9 if (b > c) c = b;
10 }
11 cout << c;
12 return 0;
13}
503
請製作一個程式,輸入一個正整數n,代表接下來共有n個整數,請輸出最大值的編號
(如果數字相同以序號小的)
1#include<iostream>
2
3using namespace std;
4int main() {
5 int i, a, b, c = -99999, d = 0;
6 cin >> a;
7 for (i = 0; i < a; i++) {
8 cin >> b;
9 if (b > c) {
10 c = b;
11 d = i;
12 }
13 }
14 cout << d;
15 return 0;
16}
504
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,利用泡沫排序法零式,將最大值移至最後方。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, i, j, b;
6 cin >> a;
7 int A[a];
8 for (i = 0; i < a; i++) {
9 cin >> j;
10 A[i] = j;
11 }
12 for (i = 0; i < a; i++) {
13 if (A[i] > A[i + 1]) {
14 b = A[i];
15 A[i] = A[i + 1];
16 A[i + 1] = b;
17 }
18 cout << A[i] << " ";
19 }
20
21 return 0;
22}
505
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,利用泡沫排序法由小到大排序。
1#include<iostream>
2
3using namespace std;
4int main() {
5 int a, i, j, b;
6 cin >> a;
7 int A[a];
8 for (i = 0; i < a; i++) {
9 cin >> j;
10 A[i] = j;
11 }
12 for (j = 0; j < a - 1; j++) {
13 for (i = 0; i < a; i++) {
14 if (A[i] > A[i + 1]) {
15 b = A[i];
16 A[i] = A[i + 1];
17 A[i + 1] = b;
18 }
19 }
20
21 }
22 for (j = 0; j < a; j++) cout << A[j] << " ";
23 return 0;
24}
506
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,利用選擇排序法零式,將最小值移至最前方。
507
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,利用選擇排序法由小到大排序。
508
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,找出中位數。
509
請製作一個程式,使用者輸入一個正整數n,代表接下來共有n個整數,找出眾數。
510
請製作一個程式,輸入一個正整數n,代表接下來共有n個整數,請檢查該數列是否等差、等比或都不是